Laravel 5.4 Basic BotDetect CAPTCHA Example
Laravel Basic BotDetect CAPTCHA Example illustrates the most basic form of BotDetect PHP Captcha protection in Laravel MVC applications.
First Time Here?
Check the BotDetect Laravel 5.4 Captcha Quickstart for key integration steps.
Alongside the Captcha image, the user is provided with an input field to retype the displayed characters. Depending on if the Captcha code entered matches the displayed one or not, a message stating the validation result is shown on the form.
The simple code showing the message in this example would of course be replaced with useful form processing code in a real world scenario.
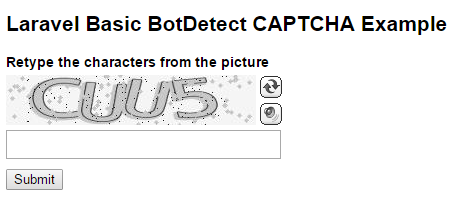
Files for this ('bd-captcha-laravel-5.4-examples') example are:
/routes/web.php
/config/captcha.php
/resources/views/example.blade.php
/app/Http/Controllers/ExampleController.php
The files are available for download as a part of the BotDetect Captcha Laravel integration package.
Routing – /routes/web.php
Route::get('example', 'ExampleController@getExample'); Route::post('example', 'ExampleController@postExample');
In the code above we have registered HTTP GET and POST verbs for the example page. On HTTP GET request to the example page, the getExample()
action of the ExampleController
is executed, while on a HTTP POST request to the example page, the postExample()
action of the ExampleController
is executed.
Config – /config/captcha.php
<?php if (!class_exists('CaptchaConfiguration')) { return; } // BotDetect PHP Captcha configuration options return [ // Captcha configuration for example page 'ExampleCaptcha' => [ 'UserInputID' => 'CaptchaCode', 'ImageWidth' => 250, 'ImageHeight' => 50, ], ];
In order to use the Laravel CAPTCHA Package, we have declared Captcha configuration which will be used when showing Captcha image in example view. Detailed description of this approach is available in a BotDetect Laravel 5.4 integration guide.
View – /resources/views/example.blade.php
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>Laravel Basic BotDetect CAPTCHA Example</title> <!-- include the BotDetect layout stylesheet --> <link href="{{ captcha_layout_stylesheet_url() }}" type="text/css" rel="stylesheet"> </head> <body> <h2>Laravel Basic BotDetect CAPTCHA Example</h2> @if (session('status')) <div class="alert alert-success"> {{ session('status') }} </div> @endif <form action="{{ url('/example') }}" method="POST"> {!! csrf_field() !!} <!-- show captcha image html --> <label>Retype the characters from the picture</label> {!! captcha_image_html('ExampleCaptcha') !!} <input type="text" id="CaptchaCode" name="CaptchaCode"> @if ($errors->has('CaptchaCode')) <span class="help-block"> <strong>{{ $errors->first('CaptchaCode') }}</strong> </span> @endif <br> <button type="submit" class="btn">Submit</button> </form> </body> </html>
The above code uses captcha_image_html()
helper function to generate Captcha image. It is required to pass a captcha configuration key defined in config/captcha.php
file. And the form also contains an input field of your choice where the user can retype the characters shown in the Captcha image. This user-entered code should be available to you in Controller code after form submission.
You should ensure that the form action points to the Controller action of the View to which it belongs to. Also, the name of the input field corresponds to the variable in the request object that we will use for Captcha validation in the Controller.
The View needs to add the required stylesheet of the library by calling captcha_layout_stylesheet_url()
helper function, and it also prints out a message about the Captcha validation status (set in the Controller).
Controller – /app/Http/Controllers/ExampleController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class ExampleController extends Controller { public function getExample() { return view('example'); } public function postExample(Request $request) { // validate the user-entered Captcha code when the form is submitted $code = $request->input('CaptchaCode'); $isHuman = captcha_validate($code); if ($isHuman) { // Captcha validation passed // TODO: continue with form processing, knowing the submission was made by a human return redirect() ->back() ->with('status', 'CAPTCHA validation passed, human visitor confirmed!'); } // Captcha validation failed, return an error message return redirect() ->back() ->withErrors(['CaptchaCode' => 'CAPTCHA validation failed, please try again.']); } }
The example Controller follows the basic instructions from the BotDetect Laravel 5.4 Captcha integration guide.
The example form submits the data to the postExample()
action, which is where we check the submitted data and pass it to the captcha_validate()
helper function.
Current BotDetect Versions
-
BotDetect ASP.NET CAPTCHA
2019-07-22v4.4.2 -
BotDetect Java CAPTCHA
2019-07-22v4.0.Beta3.7 -
BotDetect PHP CAPTCHA
2019-07-22v4.2.5