Laravel 5.7 Form Validation BotDetect CAPTCHA Example
First Time Here?
Check the BotDetect Laravel 5.7 Captcha Quickstart for key integration steps.
Laravel Form Validation BotDetect CAPTCHA Example shows how to integrate Laravel CAPTCHA validation rule and Laravel validation functionality. It uses Laravel's validation class
The brief example is based around a contact form which sends email if the user input is considered valid – a likely real world scenario for Captcha protection integration.
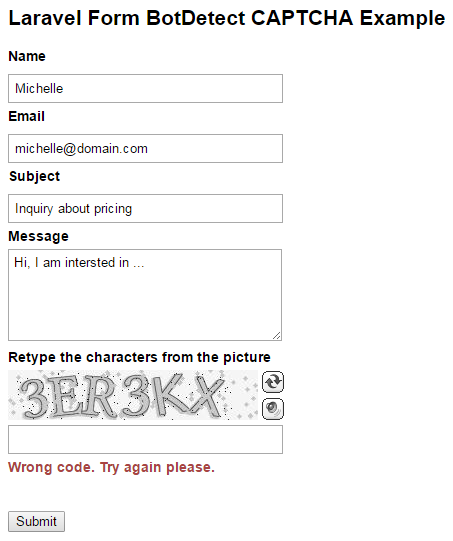
Files for this ('bd-captcha-laravel-5.7-examples') example are:
/routes/web.php
/config/captcha.php
/resources/views/contact.blade.php
/app/Http/Controllers/ContactController.php
The files are available for download as a part of the BotDetect Captcha Laravel integration package.
Routing – /routes/web.php
Route::get('contact', 'ContactController@getContact'); Route::post('contact', 'ContactController@postContact');
In the code above we have registered HTTP GET and POST verbs for the contact page. On HTTP GET request to the contact page, the getContact()
action of the ContactController
is executed, while on a HTTP POST request to the example page, the postContact()
action of the ContactController
is executed.
Config – /config/captcha.php
<?php if (!class_exists('CaptchaConfiguration')) { return; } // BotDetect PHP Captcha configuration options return [ // Captcha configuration for contact page 'ContactCaptcha' => [ 'UserInputID' => 'CaptchaCode', 'CodeLength' => CaptchaRandomization::GetRandomCodeLength(4, 6), 'ImageStyle' => ImageStyle::AncientMosaic, ], ];
In order to use the Laravel CAPTCHA Package, we have declared Captcha configuration which will be used when showing Captcha image in contact view. Detailed description of this approach is available in a BotDetect Laravel 5.7 integration guide.
View – /resources/views/contact.blade.php
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>Laravel Form BotDetect CAPTCHA Example</title> <!-- include the BotDetect layout stylesheet --> <link href="{{ captcha_layout_stylesheet_url() }}" type="text/css" rel="stylesheet"> </head> <body> <h2>Laravel Form BotDetect CAPTCHA Example</h2> @if (session('status')) <div class="alert alert-success"> {{ session('status') }} </div> @endif <form action="{{ url('/contact') }}" method="POST"> {!! csrf_field() !!} <label>Name</label> <input type="text" name="name" value="{{ old('name') }}" class="txt-input"> @if ($errors->has('name')) <span class="help-block"> <strong>{{ $errors->first('name') }}</strong> </span> @endif <label>Email</label> <input type="text" name="email" value="{{ old('email') }}" class="txt-input"> @if ($errors->has('email')) <span class="help-block"> <strong>{{ $errors->first('email') }}</strong> </span> @endif <label>Subject</label> <input type="text" name="subject" value="{{ old('subject') }}" class="txt-input"> @if ($errors->has('subject')) <span class="help-block"> <strong>{{ $errors->first('subject') }}</strong> </span> @endif <label>Message</label> <textarea name="message" class="txt-area">{{ old('message') }}</textarea> @if ($errors->has('message')) <span class="help-block"> <strong>{{ $errors->first('message') }}</strong> </span> @endif <!-- show captcha image html --> <label>Retype the characters from the picture</label> {!! captcha_image_html('ContactCaptcha') !!} <input type="text" id="CaptchaCode" name="CaptchaCode"> @if ($errors->has('CaptchaCode')) <span class="help-block"> <strong>{{ $errors->first('CaptchaCode') }}</strong> </span> @endif <br> <button type="submit" class="btn">Submit</button> </form> </body> </html>
The View part of this example is straightforward. The above code uses captcha_image_html()
helper function to generate Captcha image. It is required to pass a captcha configuration key defined in config/captcha.php
file.
The View needs to add the required stylesheet of the library by calling captcha_layout_stylesheet_url()
helper function.
Controller – /app/Http/Controllers/ContactController.php
<?php namespace App\Http\Controllers; use Validator; use Illuminate\Http\Request; class ContactController extends Controller { // get a validator for an incoming contact request. public function validator(array $data) { // custom error message for valid_captcha validation rule $messages = [ 'valid_captcha' => 'Wrong code. Try again please.' ]; return Validator::make($data, [ 'name' => 'required|min:5', 'email' => 'required|email', 'subject' => 'required|min:10', 'message' => 'required|min:20', 'CaptchaCode' => 'required|valid_captcha' ], $messages); } public function getContact() { return view('contact'); } public function postContact(Request $request) { $validator = $this->validator($request->all()); if ($validator->fails()) { return redirect() ->back() ->withInput() ->withErrors($validator->errors()); } // Captcha validation passed // TODO: send email return redirect() ->back() ->with('status', 'Your message was sent successfully.'); } }
The Controller part of the example provides necessary helpers and data used by View, and adds the Captcha validation functionality as outlined in the BotDetect Laravel 5.7 integration guide.
On HTTP POST request (which occurs when user submits the form), the postContact()
action is executed and this is where we validate the user's Captcha code input by using the valid_captcha
validation rule.
Finally, we redirect user to contact page and pass some data to contact view.
Current BotDetect Versions
-
BotDetect ASP.NET CAPTCHA
2019-07-22v4.4.2 -
BotDetect Java CAPTCHA
2019-07-22v4.0.Beta3.7 -
BotDetect PHP CAPTCHA
2019-07-22v4.2.5