CodeIgniter 3 Basic BotDetect CAPTCHA Example
This code example illustrates the most basic form of BotDetect PHP Captcha protection in CodeIgniter MVC applications.
First Time Here?
Check the BotDetect CodeIgniter 3 Captcha Quickstart for key integration steps.
Alongside the Captcha image, the user is provided with an input field to retype the displayed characters. Depending on if the Captcha code entered matches the displayed one or not, a message stating the validation result is shown on the form.
The simple code showing the message in this example would of course be replaced with useful form processing code in a real world scenario.
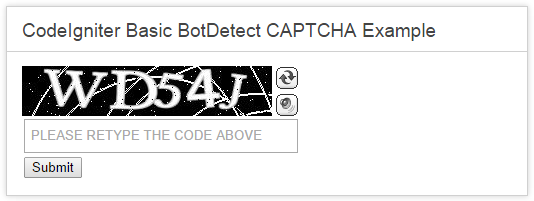
Files for this ('codeigniter_form_validation_captcha_example') example are:
/application/config/routes.php
/application/config/captcha.php
/application/views/botdetect/example.php
/application/controllers/Example.php
The files are available for download as a part of the BotDetect Captcha CodeIgniter integration package.
Routing – /application/config/routes.php
$route['botdetect/captcha-handler'] = 'botdetect/captcha_handler/index';
In the code above we have registered default route of the Captcha library.
Config – /application/config/captcha.php
<?php // BotDetect PHP Captcha configuration options $config = array( // Captcha configuration for example page 'ExampleCaptcha' => array( 'UserInputID' => 'CaptchaCode', 'ImageWidth' => 250, 'ImageHeight' => 50, ), );
In order to use the BotDetect CAPTCHA CodeIgniter Library, we have declared Captcha configuration which will be used when loading the library in ExampleController. Detailed description of this approach is available in a BotDetect CodeIgniter 3 integration guide.
View – /application/views/botdetect/example.php
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>CodeIgniter Basic BotDetect CAPTCHA Example</title> <link type="text/css" rel="Stylesheet" href="<?php echo CaptchaUrls:: LayoutStylesheetUrl() ?>" /> <link type="text/css" rel="Stylesheet" href="<?php echo base_url(); ?> css/style.css" /> </head> <body> <div id="container"> <h1>CodeIgniter Basic BotDetect CAPTCHA Example</h1> <div id="body"> <?php echo form_open('example'); ?> <label for="CaptchaCode">Please retype the characters from the image: </label> <?php echo $captchaHtml; ?> <input type="text" name="CaptchaCode" id="CaptchaCode" value="" size="50" /> <div><input type="submit" value="Submit" /></div> <?php echo $captchaValidationMessage; ?> </form> </div> </div> </body> </html>
The basic form is created through some basic Html, although the actual form can be created by using CodeIgniter form helpers. The only requirement is that your form contains an additional input field of your choice where the user can retype the characters shown in the Captcha image. This user-entered code should be available to you in Controller code after form submission.
Note that the action of the form points to the same action of the Controller the View belongs to. Also, the name of the input field corresponds to the variable in the input object that we will use for validation in the Controller.
The Captcha markup made available in the Controller is used in the View to compose a simple form with one input field and a Captcha image. The View utilizes a BotDetect supplied Css helper method to add the required stylesheet. Finally, it prints out a message about the Captcha validation status (set in the Controller).
Controller – /application/controllers/Example.php
<?php if ( ! defined('BASEPATH')) exit('No direct script access allowed'); class Example extends CI_Controller { public function index() { $this->load->helper(array('form', 'url')); // load the BotDetect Captcha library and set its parameter $this->load->library('botdetect/BotDetectCaptcha', array( 'captchaConfig' => 'ExampleCaptcha' )); // make Captcha Html accessible to View code $data['captchaHtml'] = $this->botdetectcaptcha->Html(); // initially, the message shown to the visitor is empty $data['captchaValidationMessage'] = ''; if ($_POST) { // validate the user-entered Captcha code when the form is submitted $code = $this->input->post('CaptchaCode'); $isHuman = $this->botdetectcaptcha->Validate($code); if ($isHuman) { // Captcha validation passed $data['captchaValidationMessage'] = 'CAPTCHA validation passed, human visitor confirmed!'; // TODO: continue with form processing, knowing the submission was made by a human } else { // Captcha validation failed, return an error message $data['captchaValidationMessage'] = 'CAPTCHA validation failed, please try again.'; } } $this->load->view('botdetect/example', $data); } }
The example Controller follows the basic instructions from the BotDetect CodeIgniter 3 guide.
After loading the required helpers and the BotDetect Captcha CodeIgniter library, the Html required for displaying the Captcha image and integrated controls is made available to the View by adding it to the $data
array.
The example form submits the data to the same Controller action that shows it (index), which is where we check the submitted data and pass it to the Validate()
method of the Captcha library object.
Current BotDetect Versions
-
BotDetect ASP.NET CAPTCHA
2019-07-22v4.4.2 -
BotDetect Java CAPTCHA
2019-07-22v4.0.Beta3.7 -
BotDetect PHP CAPTCHA
2019-07-22v4.2.5