How To Add BotDetect CAPTCHA Protection to Struts Forms
Protecting your Struts forms with BotDetect Java Captcha requires a few steps, outlined on this page.
You can also see how BotDetect Captcha protection has been added to various kinds of Struts forms and projects by running the BotDetect Captcha Struts integration code examples coming with the BotDetect installation. You can also reuse the code example source code that fits your requirements.
CAPTCHA Integration Steps
To add BotDetect Captcha protection to a Struts application:
- Add BotDetect library in the classpath
- Register
CaptchaServlet
- Display Captcha protection on the Struts form
- Validate Captcha user input during Struts form submission
Add BotDetect Java CAPTCHA Library Dependency
Here is how to add BotDetect Java CAPTCHA Library dependency in various dependency management scenarios:
Install BotDetect Java CAPTCHA dependencies
The free version Maven artifacts are available from our public repository; while the enterprise version jars are available in the root folder of the enterprise version's archive.
To reference the BotDetect dependency from our public repository, the repository itself has to be declared first -- add the highlighted lines
to your app's pom.xml
file:
<repository> <id>captcha</id> <name>BotDetect Captcha Repository</name> <url>https://git.captcha.com/botdetect-java-captcha.git/blob_plain/HEAD:/</url> </repository>
Then, in the same file, declare the BotDetect dependency, too:
<dependency> <groupId>com.captcha</groupId> <artifactId>botdetect-jsp20</artifactId> <version>4.0.beta3.7</version> </dependency>
Register CaptchaServlet
Update your application configuration (web.xml
) file.
<servlet> <servlet-name>BotDetect Captcha</servlet-name> <servlet-class>com.captcha.botdetect.web.servlet.CaptchaServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>BotDetect Captcha</servlet-name> <url-pattern>/botdetectcaptcha</url-pattern> </servlet-mapping>
Display Captcha Protection on the Struts Form
We'll assume you already have a form which can be posted (<form method="post" ...
), with other fields in place.
BotDetect custom captcha
tag is designed to add CAPTCHA protection to Struts form as simple as possible.
At the top of the file put BotDetect taglib
declaration:
<%@taglib prefix="botDetect" uri="https://captcha.com/java/jsp"%>
To display the Captcha test on your form, you will need the following Html elements:
- A textbox for the Captcha code user input, with a label displaying Captcha instructions
- The Captcha markup including the image, sound and reload icons etc., which will be generated by the Captcha tag.
For example:
<botDetect:captcha id="exampleCaptcha" userInputID="captchaCode"/> <div class="validationDiv"> <input id="captchaCode" type="text" name="captchaCode" value="${basicExample.captchaCode}"/> <input type="submit" name="submit" value="Submit" /> <span class="correct">${basicExample.captchaCorrect}</span> <span class="incorrect">${basicExample.captchaIncorrect}</span> </div>
And you also need to add exclude directive for CaptchaServlet
to Struts 2 filter by adding the following in your
struts.xml
configuration file:
<constant name="struts.action.excludePattern" value="/botdetectcaptcha"/>
When you open your form in a browser, the above declarations should render as:
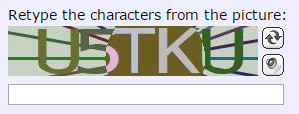
If you are adding Captcha protection to multiple Struts forms in the same website, you should initialize each Captcha
object with unique name (e.g. "registrationCaptcha"
, "commentCaptcha"
, ...).
Validate Captcha User Input During Struts Form Submission
Since we want to ensure only real human users can perform a certain action (e.g. account registration or comment submission), we also have to add Captcha validation code which will process form submissions, and only allow certain actions if Captcha validation succeeds.
Add CAPTCHA Validation Logic to Struts Action
When the form is submitted, the Captcha validation result must be checked and the protected action (user registration, comment posting, email sending, ...) only performed if the Captcha test was passed. You just have to create Captcha
object instance with the same name as the one used on the form first. For example, this code should be part of or invoked from Struts action's validate
method:
[...] public class ExampleCaptchaAction extends ActionSupport { private String captchaCode; public String getCaptchaCode() { return captchaCode; } public void setCaptchaCode(String captchaCode) { this.captchaCode = captchaCode; } public String execute() { return SUCCESS; } public void validate() { Captcha captcha = Captcha.load(ServletActionContext.getRequest(), "exampleCaptcha"); boolean isHuman = captcha.validate(captchaCode); if (!isHuman) { addFieldError("captchaCode", "Incorrect code"); } } }
This approach is shown in the BotDetect Struts Basic CAPTCHA integration code example included in the BotDetect download package.
Please Note
BotDetect Java Captcha Library v4.0.Beta3.7 is an in-progress port of BotDetect 4 Captcha, and we need you to guide our efforts towards a polished product. Please let us know if you encounter any bugs, implementation issues, or a usage scenario you would like to discuss.
Current BotDetect Versions
-
BotDetect ASP.NET CAPTCHA
2019-07-22v4.4.2 -
BotDetect Java CAPTCHA
2019-07-22v4.0.Beta3.7 -
BotDetect PHP CAPTCHA
2019-07-22v4.2.5