How To Add BotDetect CAPTCHA Protection to Spring 5 Forms
Protecting your Spring MVC 5 forms with BotDetect Java Captcha requires a few steps, outlined on this page.
You can also see how BotDetect Captcha protection has been added to various kinds of Spring MVC forms and projects by running the BotDetect Captcha Spring integration code examples coming with the BotDetect installation. You can also reuse the code example source code that fits your requirements.
CAPTCHA Integration Steps
To add BotDetect Captcha protection to a Spring MVC application:
- Add BotDetect library in the classpath
- Register
CaptchaServlet
- Display Captcha protection on the Spring MVC form
- Validate Captcha user input during Spring MVC form submission
Add BotDetect Java CAPTCHA Library Dependency
Here is how to add BotDetect Java CAPTCHA Library dependency in various dependency management scenarios:
Install BotDetect Java CAPTCHA dependencies
The free version Maven artifacts are available from our public repository; while the enterprise version jars are available in the root folder of the enterprise version's archive.
To reference the BotDetect dependency from our public repository, the repository itself has to be declared first -- add the highlighted lines
to your app's pom.xml
file:
<repository> <id>captcha</id> <name>BotDetect Captcha Repository</name> <url>https://git.captcha.com/botdetect-java-captcha.git/blob_plain/HEAD:/</url> </repository>
Then, in the same file, declare the BotDetect dependency, too:
<dependency> <groupId>com.captcha</groupId> <artifactId>botdetect-jsp20</artifactId> <version>4.0.beta3.7</version> </dependency>
Register CaptchaServlet
Application web.xml
configuration file setting
<servlet> <servlet-name>BotDetect Captcha</servlet-name> <servlet-class>com.captcha.botdetect.web.servlet.CaptchaServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>BotDetect Captcha</servlet-name> <url-pattern>/botdetectcaptcha</url-pattern> </servlet-mapping>
Spring Boot servlet registration
In case you are using Spring Boot servlet is registered in a following way:
@SpringBootApplication public class SpringBootWebApplication { [...] @Bean ServletRegistrationBean captchaServletRegistration () { ServletRegistrationBean srb = new ServletRegistrationBean(); srb.setServlet(new CaptchaServlet()); srb.addUrlMappings("/botdetectcaptcha"); return srb; } }
Display Captcha Protection on the Spring MVC Form
We'll assume you already have a form which can be posted (<form method="post" ...
), with other fields in place.
BotDetect custom captcha
tag is designed to add CAPTCHA protection to Spring MVC form as simple as possible.
At the top of the file put BotDetect taglib
declaration:
<%@taglib prefix="botDetect" uri="https://captcha.com/java/jsp"%>
To display the Captcha test on your form, you will need the following Html elements:
- A textbox for the Captcha code user input, with a label displaying Captcha instructions
- The Captcha markup including the image, sound and reload icons etc., which will be generated by the Captcha tag.
For example:
<botDetect:captcha id="exampleCaptcha" userInputID="captchaCode"/> <div class="validationDiv"> <input id="captchaCode" type="text" name="captchaCode" value="${basicExample.captchaCode}"/> <input type="submit" name="submit" value="Submit" /> <span class="correct">${basicExample.captchaCorrect}</span> <span class="incorrect">${basicExample.captchaIncorrect}</span> </div>
When you open your form in a browser, the above declarations should render as:
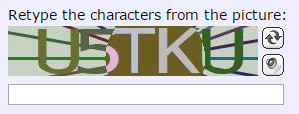
If you are adding Captcha protection to multiple Spring MVC forms in the same website, you should initialize each Captcha
object with unique name (e.g. "registrationCaptcha"
, "commentCaptcha"
, ...).
Validate Captcha User Input During Spring MVC Form Submission
Since we want to ensure only real human users can perform a certain action (e.g. account registration or comment submission), we also have to add Captcha validation code which will process form submissions, and only allow certain actions if Captcha validation succeeds.
Add CAPTCHA Validation Logic to Spring MVC Controller
When the form is submitted, the Captcha validation result must be checked and the protected action (user registration, comment posting, email sending, ...) only performed if the Captcha test was passed. You just have to create Captcha
object instance with the same name as the one used on the form first. For example, this code should be part of or invoked from controller's onSubmit
method:
[...] import com.captcha.botdetect.web.servlet.Captcha; @Controller public class ExampleController { [...] @RequestMapping(value = "/validate", method = RequestMethod.POST) public ModelAndView onSubmit(HttpServletRequest request, @Valid @ModelAttribute("example")BasicExample basicExample) { // validate the Captcha to check we're not dealing with a bot Captcha captcha = Captcha.load(request, "exampleCaptcha"); boolean isHuman = captcha.validate(basicExample.getCaptchaCode()); if (isHuman) { // TODO: Captcha validation passed, perform protected action } else { // TODO: Captcha validation failed, show error message } return new ModelAndView("example", "basicExample", basicExample); } }
This approach is shown in the BotDetect Spring MVC 5 Basic CAPTCHA integration code example included in the BotDetect download package.
Please Note
BotDetect Java Captcha Library v4.0.Beta3.7 is an in-progress port of BotDetect 4 Captcha, and we need you to guide our efforts towards a polished product. Please let us know if you encounter any bugs, implementation issues, or a usage scenario you would like to discuss.
Current BotDetect Versions
-
BotDetect ASP.NET CAPTCHA
2019-07-22v4.4.2 -
BotDetect Java CAPTCHA
2019-07-22v4.0.Beta3.7 -
BotDetect PHP CAPTCHA
2019-07-22v4.2.5