CakePHP 3.0 Basic BotDetect CAPTCHA Example (BotDetect v3.0; deprecated)
This code example illustrates the most basic form of BotDetect PHP Captcha protection in CakePHP MVC applications.
First Time Here?
Check the BotDetect CakePHP 3.0 Captcha Quickstart for key integration steps.
Alongside the Captcha image, the user is provided with an input field to retype the displayed characters. Depending on if the Captcha code entered matches the displayed one or not, a message stating the validation result is shown on the form.
The simple code showing the message in this example would of course be replaced with useful form processing code in a real world scenario.
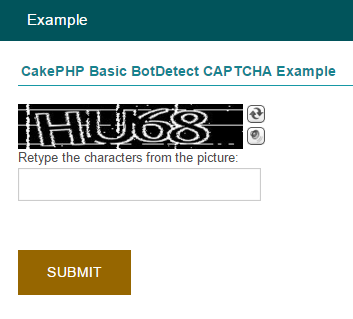
Files for this ('bd-captcha-cakephp-3.0-examples') example are:
/src/Template/Example/index.ctp
/src/Controller/ExampleController.php
/config/captcha_config/ExampleCaptchaConfig.php
The files are available for download as a part of the BotDetect Captcha CakePHP integration package.
View – /src/Template/Example/index.ctp
<!-- include the BotDetect layout stylesheet --> <?= $this->Html->css(CaptchaUrls::LayoutStylesheetUrl(), array('inline' => false)) ?> <div class="users form"> <?= $this->Form->create(null, array('action' => 'index')) ?> <fieldset> <legend><?= __('CakePHP Basic BotDetect CAPTCHA Example') ?></legend> <!-- show captcha image --> <?= $captchaHtml ?> <!-- Captcha code user input textbox --> <?= $this->Form->input('CaptchaCode', array( 'label' => 'Retype the characters from the picture:', 'maxlength' => '10', 'style' => 'width: 270px;', 'id' => 'CaptchaCode' ) ) ?> </fieldset> <?= $this->Form->button(__('Submit'), ['style' => 'float: left']) ?> <?= $this->Form->end() ?> </div>
The above code uses the CakePHP FormHelper
to generate a form, although the actual form can be created in many other ways. The only requirement is that the form contains an input field of your choice where the user can retype the characters shown in the Captcha image. This user-entered code should be available to you in Controller code after form submission.
Note that the action of the form points to the same action of the Controller the View belongs to. Also, the name of the input field corresponds to the variable in the request object that we will use for validation in the Controller.
The helpers and the Captcha markup made available in the Controller are used in the View to compose a simple form with one input field and a Captcha image. The View utilizes the HtmlHelper::css()
method to add the required stylesheet, and it also prints out a message about the Captcha validation status (set in the Controller).
Controller – /src/Controller/ExampleController.php
<?php namespace App\Controller; use App\Controller\AppController; // Importing the BotDetectCaptcha class use CakeCaptcha\Integration\BotDetectCaptcha; class ExampleController extends AppController { // get captcha instance to handle for the example page private function getExampleCaptchaInstance() { // Captcha parameters $captchaConfig = [ 'CaptchaId' => 'ExampleCaptcha', // a unique Id for the Captcha instance 'UserInputId' => 'CaptchaCode', // Id of the Captcha code input textbox // The path of the Captcha config file is inside the config folder 'CaptchaConfigFilePath' => 'captcha_config/ExampleCaptchaConfig.php', ]; return BotDetectCaptcha::GetCaptchaInstance($captchaConfig); } public function index() { // captcha instance of the example page $captcha = $this->getExampleCaptchaInstance(); // passing Captcha Html to example view $this->set('captchaHtml', $captcha->Html()); if ($this->request->is('post')) { // validate the user-entered Captcha code $isHuman = $captcha->Validate($this->request->data['CaptchaCode']); // clear previous user input, since each Captcha code can only be validated once unset($this->request->data['CaptchaCode']); if ($isHuman) { // Captcha validation passed $this->Flash->success(__('CAPTCHA validation passed, human visitor confirmed!')); // TODO: continue with form processing, knowing the submission // was made by a human } else { // Captcha validation failed, return an error message $this->Flash->error(__('CAPTCHA validation failed, please try again.')); } } } }
The example Controller follows the basic instructions from the BotDetect CakePHP 3.0 integration guide.
First, we need to create a function to get an instance of the Captcha class called getExampleCaptchaInstance()
, and then the Html required for displaying the Captcha image and integrated controls is made available to the View by using the Controller:set()
method.
The example form submits the data to the same Controller action that shows it (index), which is where we check the submitted data and pass it to the Validate()
method of the Captcha object.
Captcha configuration options – /config/captcha_config/ExampleCaptchaConfig.php
<?php if (!class_exists('CaptchaConfiguration')) { return; } // BotDetect PHP Captcha configuration options $LBD_CaptchaConfig = CaptchaConfiguration::GetSettings(); $LBD_CaptchaConfig->CodeLength = 5; $LBD_CaptchaConfig->ImageWidth = 250; $LBD_CaptchaConfig->ImageHeight = 50;
In the code above, we have overridden the default settings of library. You can find a full list of available Captcha configuration options and related instructions at the Captcha configuration options page.
Please Note
The information on this page is out of date and applies to a deprecated version of BotDetect™ CAPTCHA (v3.0).
An up-to-date equivalent page for the latest BotDetect Captcha release (v4) is BotDetect v4 Captcha documentation index.
General information about the major improvements in the current BotDetect release can be found at the What's New in BotDetect v4.0 page.
Current BotDetect Versions
-
BotDetect ASP.NET CAPTCHA
2019-07-22v4.4.2 -
BotDetect Java CAPTCHA
2019-07-22v4.0.Beta3.7 -
BotDetect PHP CAPTCHA
2019-07-22v4.2.5