How To Add BotDetect CAPTCHA Protection to ASP.NET Web Pages Applications
Captcha
class, implemented in the BotDetect.dll assembly. Displaying the Captcha challenge can be as simple as:
Captcha exampleCaptcha = new Captcha("ExampleCaptcha");and checking user input when the form is submitted:
bool isHuman = exampleCaptcha.Validate();
First Time Here?
Check the BotDetect ASP.NET Web Pages Captcha Quickstart for key integration steps.
You can reuse the example projects source code (both C# and VB.NET are available) that fits your application requirements.
CAPTCHA Integration Steps
When adding BotDetect Captcha protection to an ASP.NET Web Pages application:
- Display a Captcha challenge on the Asp.Net Web Pages page
- Check is the visitor a human on ASP.NET Web Pages page
- Further Captcha customization and options
I. Display a Captcha challenge on the Asp.Net Web Pages page
Before protecting a form action in your ASP.NET Web Pages application with BotDetect Captcha, you should decide how to call the Captcha instance and the related textbox you will use. In this guide, we will use ExampleCaptcha
and CaptchaCode
. If you plan to protect multiple pages within the same ASP.NET Web Pages application, you should take care to give each Captcha instance a different name (e.g. LoginCaptcha
, RegisterCaptcha
, CommentCaptcha
etc.).
You will also need to register a HttpHandler
that will handle Captcha requests.
Reference the BotDetect.dll
Assembly
Run the following command in the Package Manager Console:
PM> Install-Package Captcha
After the command execution, the references to the BotDetect.dll
, BotDetect.Web.Mvc.dll
, and
System.Data.SQLite.dll
assemblies will be added to your project.
If your project is not going to be using the Simple API, the System.Data.SQLite.dll
assembly reference can be safely removed from it.
Create and render a BotDetect.Web.Captcha
instance
Captcha objects in ASP.NET Web Pages applications are represented by the Captcha
class. To use them, your View should first import the BotDetect.Web
namespace.
Then, you can add a Captcha challenge to the View by rendering a Html.Label
with the Captcha instructions for the user, a Html.Raw
with the string to interpret as HTML markup that serves as a container for Captcha challenges from Captcha
object instance, and a Html.TextBox
in which the user is to type the Captcha code:
@using BotDetect.Web; […] @Html.Label("Retype the code from the picture:", "CaptchaCode") @{ Captcha exampleCaptcha = new Captcha("ExampleCaptcha"); exampleCaptcha .UserInputID = "CaptchaCode"; } @Html.Raw(exampleCaptcha.Html) @Html.TextBox("CaptchaCode")
Register the BotDetect HttpHandler
for CAPTCHA Requests
BotDetect uses a special HttpHandler
for Captcha requests (Captcha images, sounds, resources...), which needs to be registered in your application before Captcha images will be displayed. This registration is a two-step process:
1. Base HttpHandler
Registration
- Locate the
<system.web> -> <httpHandlers>
section of theweb.config
file. -
Add the following BotDetect handler registration to this section:
<!-- Register the HttpHandler used for BotDetect Captcha requests --> <add verb="GET" path="BotDetectCaptcha.ashx" type="BotDetect.Web.CaptchaHandler, BotDetect"/>
2. IIS 7.0+ HttpHandler
Registration
- Locate the
<system.webServer> -> <handlers>
section of theweb.config
file. -
Add the following BotDetect handler registration to this section:
<!-- Register the HttpHandler used for BotDetect Captcha requests (IIS 7.0+) --> <remove name="BotDetectCaptchaHandler"/> <add name="BotDetectCaptchaHandler" preCondition="integratedMode" verb="GET" path="BotDetectCaptcha.ashx" type="BotDetect.Web.CaptchaHandler, BotDetect"/>
Once all of these steps have been performed, the Captcha should be displayed when you open your form in a browser:
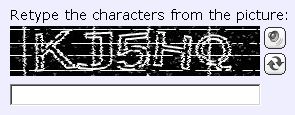
If the Captcha image isn't being rendered properly, please check the BotDetect integration FAQ.
II. Check is the visitor a human on ASP.NET Web Pages page
Once the Captcha challenge is displayed on your form, the code processing form submissions can check if the Captcha was solved successfully and deny access to bots.
This code needs to access the Captcha and textbox control instances added to the form; ASP.NET Session state must also be enabled before Captcha validation can work.
Add Captcha Validation logic to Web Pages page
When the form is submitted, the Captcha validation result must be checked and the protected action (user registration, comment posting, email sending, ...) only performed if the Captcha test was passed. For example:
@if ((Request.HttpMethod == "POST")) { bool isHuman = sampleCaptcha.Validate(); if (isHuman) { <span class="correct">Correct!</span> } else { <span class="incorrect">Incorrect!</span> } }
Configure ASP.NET Session State
BotDetect Captcha validation requires ASP.NET Session state – generated random Captcha codes have to be stored on the server and compared to the user input. Also, to ensure Captcha sounds work properly in all browsers, a custom SessionIDManager
(implementing an optional but recommended improvement) should be registered.
- In the
<system.web>
section of theweb.config
file, locate the<pages>
element and set theenableSessionState
attribute:<pages enableSessionState="true">
- In the
<system.web>
section of theweb.config
file, locate the<sessionState>
element if it exists, or add it if it doesn't. -
Add the following attribute to the declaration:
sessionIDManagerType="BotDetect.Web.CustomSessionIdManager, BotDetect"
<sessionState mode="InProc" cookieless="AutoDetect" timeout="20" sessionIDManagerType="BotDetect.Web.CustomSessionIdManager, BotDetect"/>
You can use a different Session State mode or options, since BotDetect works with all of them. For more information about BotDetect and ASP.NET Session state, and debugging tips in case the Captcha validation is failing even when you type the correct Captcha code, please check the BotDetect persistence options FAQ.
III. Further CAPTCHA Customization and Options
BotDetect ASP.NET Captcha allows detailed customization of many Captcha properties, and you can read more about them in the BotDetect Captcha Options Configuration How To.
Current BotDetect Versions
-
BotDetect ASP.NET CAPTCHA
2019-07-22v4.4.2 -
BotDetect Java CAPTCHA
2019-07-22v4.0.Beta3.7 -
BotDetect PHP CAPTCHA
2019-07-22v4.2.5