Laravel 5.0 Auth CAPTCHA Example (BotDetect v3.0; deprecated)
Laravel Auth CAPTCHA Example demonstrates how to integrate Laravel Auth is used to authenticate users to your application.
First Time Here?
Check the BotDetect Laravel 5.0 Captcha Quickstart for key integration steps.
Alongside the Captcha image, the user is provided with an input field to retype the displayed characters. Depending on if the Captcha code entered matches the displayed one or not, a message stating the validation result is shown on the form.
The simple code showing the message in this example would of course be replaced with useful form processing code in a real world scenario.
The default in Laravel 5.0 ships an example to authenticate users that is used Laravel Auth, but no captcha image is created. Here's what we started:
First, we need to configure database connection with entering connection details into the /config/database.php
file.
Next, we need to create users and password_resets tables -- using Migrations.
To create that tables, run the following command in your application's root directory:
Files for this example are:
/app/Http/routes.php
/resources/views/app.blade.php
/resources/views/auth/login.blade.php
/resources/views/auth/register.blade.php
/resources/views/auth/password.blade.php
/app/Http/Controllers/Auth/AuthController.php
/app/Http/Controllers/Auth/PasswordController.php
/config/captcha_config/LoginCaptchaConfig.php
/config/captcha_config/RegisterCaptchaConfig.php
/config/captcha_config/ResetPasswordCaptchaConfig.php
The files are available for download as a part of the BotDetect Captcha Laravel integration package.
Routing – /app/Http/routes.php
Route::controllers([ 'auth' => 'Auth\AuthController', 'password' => 'Auth\PasswordController', ]);
In the code above we use the Implicit Controllers to define a single route which will handle every action in the AuthController
and PasswordController
.
View – /resources/views/app.blade.php
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Laravel Auth Example</title> <link href="{{ URL::asset('css/bootstrap.min.css') }}" type="text/css" rel="stylesheet"> <!-- include the BotDetect layout stylesheet --> @if (class_exists('CaptchaUrls')) <link href="{{ CaptchaUrls::LayoutStylesheetUrl() }}" type="text/css" rel="stylesheet"> @endif </head> <body> <nav class="navbar navbar-default"> <div class="container-fluid"> <div class="navbar-header"> <button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#bs-example-navbar-collapse-1"> <span class="sr-only">Toggle Navigation</span> <span class="icon-bar"></span> <span class="icon-bar"></span> <span class="icon-bar"></span> </button> <a class="navbar-brand" href="#">Laravel Auth</a> </div> <div class="collapse navbar-collapse" id="bs-example-navbar-collapse-1"> <ul class="nav navbar-nav"> <li><a href="/">Home</a></li> </ul> <ul class="nav navbar-nav navbar-right"> @if (Auth::guest()) <li><a href="{{ URL::to('auth/login') }}">Login</a></li> <li><a href="{{ URL::to('auth/register') }}">Register</a></li> @else <li class="dropdown"> <a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-expanded="false"> {{ Auth::user()->name }} <span class="caret"></span> </a> <ul class="dropdown-menu" role="menu"> <li><a href="{{ URL::to('auth/logout') }}">Logout</a></li> </ul> </li> @endif </ul> </div> </div> </nav> @yield('content') <!-- Scripts --> <script src="//cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script> <script src="//cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.1/js/bootstrap.min.js"></script> </body> </html>
The above code is defining a Blade Layout. The app view needs to add the required stylesheet of the library.
View – /resources/views/auth/login.blade.php
@extends('app') @section('content') <div class="container-fluid"> <div class="row"> <div class="col-md-8 col-md-offset-2"> <div class="panel panel-default"> <div class="panel-heading">Login</div> <div class="panel-body"> @if (count($errors) > 0) <div class="alert alert-danger"> <strong>Whoops!</strong> There were some problems with your input .<br><br> <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif <form class="form-horizontal" role="form" method="POST" action="{{ URL::to('auth/login') }}"> <input type="hidden" name="_token" value="{{ csrf_token() }}"> <div class="form-group"> <label class="col-md-4 control-label">E-Mail Address</label> <div class="col-md-6"> <input type="email" class="form-control" name="email" value="{{ old('email') }}"> </div> </div> <div class="form-group"> <label class="col-md-4 control-label">Password</label> <div class="col-md-6"> <input type="password" class="form-control" name="password"> </div> </div> <div class="form-group"> <div class="col-md-6 col-md-offset-4"> <!-- Captcha image html--> {!! $captchaHtml !!} </div> <div class="col-md-6 col-md-offset-4"> <!-- Captcha code user input textbox --> <input type="text" class="form-control" id="CaptchaCode" name="CaptchaCode" style="width: 276px; margin-top: 5px"> </div> </div> <div class="form-group"> <div class="col-md-6 col-md-offset-4"> <div class="checkbox"> <label> <input type="checkbox" name="remember"> Remember Me </label> </div> </div> </div> <div class="form-group"> <div class="col-md-6 col-md-offset-4"> <button type="submit" class="btn btn-primary" style="margin-right: 15px;"> Login </button> <a href="{{ URL::to('password/email') }}">Forgot Your Password?</a> </div> </div> </form> </div> </div> </div> </div> </div> @endsection
The above code to display authentication in a view, we output the variable $captchaHtml
(set in, and passed by in the getLogin()
action of the AuthController
).
We have added Captcha Code input field to view. This Captcha Code input will be checked in the postLogin()
action later.
Login page screenshot:
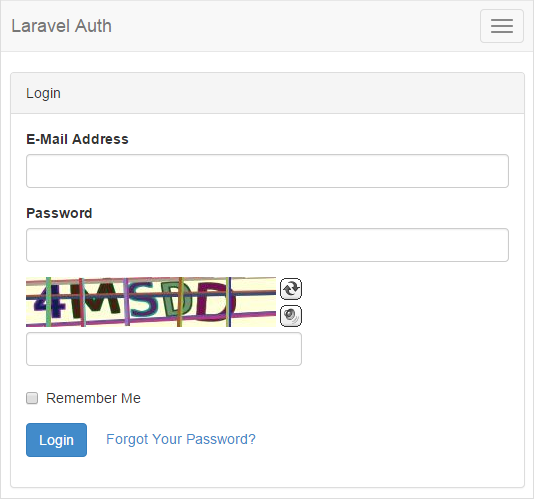
View – /resources/views/auth/register.blade.php
@extends('app') @section('content') <div class="container-fluid"> <div class="row"> <div class="col-md-8 col-md-offset-2"> <div class="panel panel-default"> <div class="panel-heading">Register</div> <div class="panel-body"> @if (count($errors) > 0) <div class="alert alert-danger"> <strong>Whoops!</strong> There were some problems with your input .<br><br> <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif <form class="form-horizontal" role="form" method="POST" action="{{ URL::to('auth/register') }}"> <input type="hidden" name="_token" value="{{ csrf_token() }}"> <div class="form-group"> <label class="col-md-4 control-label">Name</label> <div class="col-md-6"> <input type="text" class="form-control" name="name" value="{{ old('name') }}"> </div> </div> <div class="form-group"> <label class="col-md-4 control-label">E-Mail Address</label> <div class="col-md-6"> <input type="email" class="form-control" name="email" value="{{ old('email') }}"> </div> </div> <div class="form-group"> <label class="col-md-4 control-label">Password</label> <div class="col-md-6"> <input type="password" class="form-control" name="password"> </div> </div> <div class="form-group"> <label class="col-md-4 control-label">Confirm Password</label> <div class="col-md-6"> <input type="password" class="form-control" name="password_confirmation"> </div> </div> <div class="form-group"> <div class="col-md-6 col-md-offset-4"> <!-- Captcha image html --> {!! $captchaHtml !!} </div> <div class="col-md-6 col-md-offset-4"> <!-- Captcha code user input textbox --> <input type="text" class="form-control" id="CaptchaCode" name="CaptchaCode" style="width: 276px; margin-top: 5px"> </div> </div> <div class="form-group"> <div class="col-md-6 col-md-offset-4"> <button type="submit" class="btn btn-primary"> Register </button> </div> </div> </form> </div> </div> </div> </div> </div> @endsection
The above code to display authentication in a view, we output the variable $captchaHtml
(set in, and passed by in the getRegister()
action of the UsersController
).
We also added Captcha Code input field to view. This Captcha Code input will be checked in the postRegister()
action later.
Register page screenshot:
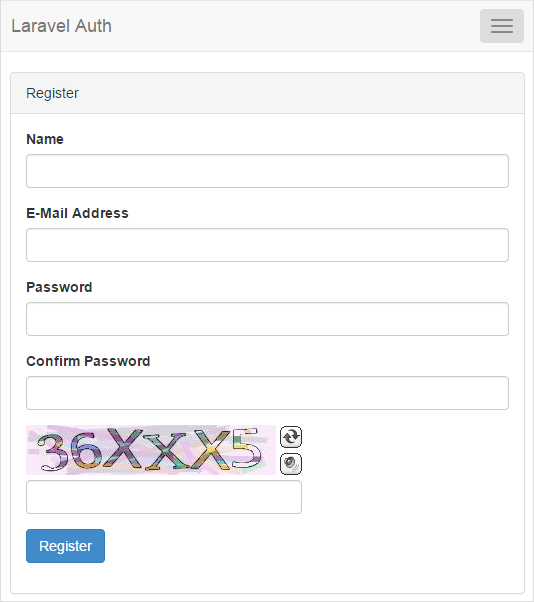
View – /resources/views/auth/password.blade.php
@extends('app') @section('content') <div class="container-fluid"> <div class="row"> <div class="col-md-8 col-md-offset-2"> <div class="panel panel-default"> <div class="panel-heading">Reset Password</div> <div class="panel-body"> @if (session('status')) <div class="alert alert-success"> {{ session('status') }} </div> @endif @if (count($errors) > 0) <div class="alert alert-danger"> <strong>Whoops!</strong> There were some problems with your input .<br><br> <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif <form class="form-horizontal" role="form" method="POST" action="{{ URL::to('password/email') }}"> <input type="hidden" name="_token" value="{{ csrf_token() }}"> <div class="form-group"> <label class="col-md-4 control-label">E-Mail Address</label> <div class="col-md-6"> <input type="email" class="form-control" name="email" value="{{ old('email') }}"> </div> </div> <div class="form-group"> <div class="col-md-6 col-md-offset-4"> <!-- Captcha image html --> {!! $captchaHtml !!} </div> <div class="col-md-6 col-md-offset-4"> <!-- Captcha code user input textbox --> <input type="text" class="form-control" id="CaptchaCode" name="CaptchaCode" style="width: 276px; margin-top: 5px"> </div> </div> <div class="form-group"> <div class="col-md-6 col-md-offset-4"> <button type="submit" class="btn btn-primary"> Send Password Reset Link </button> </div> </div> </form> </div> </div> </div> </div> </div> @endsection
The above code to display authentication in a view, we output the variable $captchaHtml
(set in, and passed by in the getEmail()
action of the PasswordController
).
We also added Captcha Code input field to view. This Captcha Code input will be checked in the postEmail()
action later.
Reset password page screenshot:
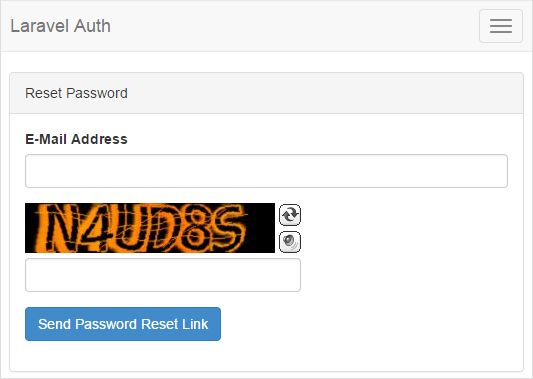
Controller – /app/Http/Controllers/Auth/AuthController.php
<?php namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use Illuminate\Contracts\Auth\Guard; use Illuminate\Contracts\Auth\Registrar; use Illuminate\Foundation\Auth\AuthenticatesAndRegistersUsers; use Illuminate\Http\Request; // Importing the BotDetectCaptcha class use LaravelCaptcha\Integration\BotDetectCaptcha; class AuthController extends Controller { /* |-------------------------------------------------------------------------- | Registration & Login Controller |-------------------------------------------------------------------------- | | This controller handles the registration of new users, as well as the | authentication of existing users. By default, this controller uses | a simple trait to add these behaviors. Why don't you explore it? | */ use AuthenticatesAndRegistersUsers; /** * Create a new authentication controller instance. * * @param \Illuminate\Contracts\Auth\Guard $auth * @param \Illuminate\Contracts\Auth\Registrar $registrar * @return void */ public function __construct(Guard $auth, Registrar $registrar) { $this->auth = $auth; $this->registrar = $registrar; $this->middleware('guest', ['except' => 'getLogout']); } // get captcha instance to handle for the register page private function getRegisterCaptchaInstance() { // Captcha parameters: $captchaConfig = [ 'CaptchaId' => 'RegisterCaptcha', // a unique Id for the Captcha instance 'UserInputId' => 'CaptchaCode', // Id of the Captcha code input textbox // The path of the Captcha config file is inside the Controllers folder 'CaptchaConfigFilePath' => 'captcha_config/RegisterCaptchaConfig.php', ]; return BotDetectCaptcha::GetCaptchaInstance($captchaConfig); } public function getRegister() { // captcha instance of the register page $captcha = $this->getRegisterCaptchaInstance(); // passing Captcha Html to register view return view('auth.register', ['captchaHtml' => $captcha->Html()]); } public function postRegister(Request $request) { $validator = $this->registrar->validator($request->all()); // captcha instance of the register page $captcha = $this->getRegisterCaptchaInstance(); // validate the user-entered Captcha code when the form is submitted $code = $request->input('CaptchaCode'); $isHuman = $captcha->Validate($code); if (!$isHuman || $validator->fails()) { if (!$isHuman) { $validator->errors()->add('CaptchaCode', 'Wrong code. Try again please.'); } return redirect() ->back() ->withInput() ->withErrors($validator->errors()); } $this->auth->login($this->registrar->create($request->all())); return redirect($this->redirectPath()); } // get captcha instance to handle for the login page private function getLoginCaptchaInstance() { // Captcha parameters: $captchaConfig = [ 'CaptchaId' => 'LoginCaptcha', // a unique Id for the Captcha instance 'UserInputId' => 'CaptchaCode', // Id of the Captcha code input textbox // The path of the Captcha config file is inside the Controllers folder 'CaptchaConfigFilePath' => 'captcha_config/LoginCaptchaConfig.php', ]; return BotDetectCaptcha::GetCaptchaInstance($captchaConfig); } public function getLogin() { // captcha instance of the login page $captcha = $this->getLoginCaptchaInstance(); // passing Captcha Html to login view return view('auth.login', ['captchaHtml' => $captcha->Html()]); } public function postLogin(Request $request) { $this->validate($request, [ 'email' => 'required|email', 'password' => 'required', 'CaptchaCode' => 'required' ]); // captcha instance of the login page $captcha = $this->getLoginCaptchaInstance(); // validate the user-entered Captcha code when the form is submitted $code = $request->input('CaptchaCode'); $isHuman = $captcha->Validate($code); $errorMessages = []; if ($isHuman) { $credentials = $request->only('email', 'password'); if ($this->auth->attempt($credentials, $request->has('remember'))) { return redirect()->intended($this->redirectPath()); } else { $errorMessages = ['email' => $this->getFailedLoginMesssage()]; } } else { $errorMessages = ['CaptchaCode' => 'Wrong code. Try again please.']; } return redirect($this->loginPath()) ->withInput($request->only('email', 'remember')) ->withErrors($errorMessages); } }
The Controller part of the example provides necessary helpers and data used by View, and adds the Captcha validation functionality as outlined in the BotDetect Laravel 5.0 integration guide.
The code above is that we are overridden the AuthenticatesAndRegistersUsers
trait of the Laravel.
After creating two functions to get an instance of the Captcha class for each page called getRegisterCaptchaInstance()
and getLoginCaptchaInstance()
, on a HTTP GET request the getRegister()
, and the getLogin()
actions pass the generated Captcha Html to View by pass an array of data as the second parameter to the view view
helper (or you can also use the with()
method).
Method: postRegister()
On HTTP POST request (user submit), the postRegister()
action executes and we validate user entered data using the Validation class and validate Captcha Code with the Validate()
method of the $captcha
object.
Method: postLogin()
On HTTP POST request (user submit), the postLogin()
action executes and we check user's email and password using the Auth::attempt()
and validate Captcha Code with the Validate()
method of the $captcha
object.
Controller – /app/Http/Controllers/Auth/PasswordController.php
<?php namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use Illuminate\Contracts\Auth\Guard; use Illuminate\Contracts\Auth\PasswordBroker; use Illuminate\Foundation\Auth\ResetsPasswords; use Illuminate\Http\Request; // Importing the BotDetectCaptcha class use LaravelCaptcha\Integration\BotDetectCaptcha; class PasswordController extends Controller { /* |-------------------------------------------------------------------------- | Password Reset Controller |-------------------------------------------------------------------------- | | This controller is responsible for handling password reset requests | and uses a simple trait to include this behavior. You're free to | explore this trait and override any methods you wish to tweak. | */ use ResetsPasswords; /** * Create a new password controller instance. * * @param \Illuminate\Contracts\Auth\Guard $auth * @param \Illuminate\Contracts\Auth\PasswordBroker $passwords * @return void */ public function __construct(Guard $auth, PasswordBroker $passwords) { $this->auth = $auth; $this->passwords = $passwords; $this->middleware('guest'); } // get captcha instance to handle for the reset password page private function getResetPasswordCaptchaInstance() { // Captcha parameters: $captchaConfig = [ 'CaptchaId' => 'ResetPasswordCaptcha', // a unique Id for the Captcha instance 'UserInputId' => 'CaptchaCode', // Id of the Captcha code input textbox // The path of the Captcha config file is inside the Controllers folder 'CaptchaConfigFilePath' => 'captcha_config/ResetPasswordCaptchaConfig.php', ]; return BotDetectCaptcha::GetCaptchaInstance($captchaConfig); } public function getEmail() { // captcha instance of the reset password page $captcha = $this->getResetPasswordCaptchaInstance(); // passing Captcha Html to reset password view return view('auth.password', ['captchaHtml' => $captcha->Html()]); } public function postEmail(Request $request) { $this->validate($request, [ 'email' => 'required|email', 'CaptchaCode' => 'required', ]); // captcha instance of the reset password page $captcha = $this->getResetPasswordCaptchaInstance(); // validate the user-entered Captcha code when the form is submitted $code = $request->input('CaptchaCode'); $isHuman = $captcha->Validate($code); $errorMessages = []; if ($isHuman) { $response = $this->passwords->sendResetLink($request->only('email'), function($m) { $m->subject($this->getEmailSubject()); }); switch ($response) { case PasswordBroker::RESET_LINK_SENT: return redirect()->back()->with('status', trans($response)); case PasswordBroker::INVALID_USER: return redirect()->back()->withErrors(['email' => trans($response)]); } } return redirect() ->back() ->withErrors(['CaptchaCode' => 'Wrong code. Try again please.']); } }
The Controller part of the example provides necessary helpers and data used by View, and adds the Captcha validation functionality as outlined in the BotDetect Laravel 5.0 integration guide.
The code above is that we are overridden the ResetsPasswords
trait of the Laravel.
After creating a function to get an instance of the Captcha class called getResetPasswordCaptchaInstance()
, on a HTTP GET request the getEmail()
action pass the generated Captcha Html to View by pass an array of data as the second parameter to the view view
helper (or you can also use the with()
method).
On HTTP POST request (user submit), the postEmail()
action executes and we validate user entered data using the Validation class and validate Captcha Code with the Validate()
method of the $captcha
object.
Captcha configuration options – /config/captcha_config/LoginCaptchaConfig.php
<?php if (!class_exists('CaptchaConfiguration')) { return; } // BotDetect PHP Captcha configuration options $LBD_CaptchaConfig = CaptchaConfiguration::GetSettings(); $imageStyles = array( ImageStyle::Chipped, ImageStyle::Negative, ); $LBD_CaptchaConfig->ImageStyle = CaptchaRandomization::GetRandomImageStyle($imageStyles);
In the code above, we have overridden the default settings of library for the login page. You can find a full list of available Captcha configuration options and related instructions at the Captcha configuration options page.
Captcha configuration options – /config/captcha_config/RegisterCaptchaConfig.php
<?php if (!class_exists('CaptchaConfiguration')) { return; } // BotDetect PHP Captcha configuration options $LBD_CaptchaConfig = CaptchaConfiguration::GetSettings(); $LBD_CaptchaConfig->CodeLength = 6; $LBD_CaptchaConfig->ImageWidth = 250; $LBD_CaptchaConfig->ImageHeight = 50;
In the code above, we have overridden the default settings of library for the register page. You can find a full list of available Captcha configuration options and related instructions at the Captcha configuration options page.
Captcha configuration options – /config/captcha_config/ResetPasswordCaptchaConfig.php
<?php if (!class_exists('CaptchaConfiguration')) { return; } // BotDetect PHP Captcha configuration options $LBD_CaptchaConfig = CaptchaConfiguration::GetSettings(); $LBD_CaptchaConfig->CodeLength = CaptchaRandomization::GetRandomCodeLength(3, 5); $LBD_CaptchaConfig->ImageWidth = 250; $LBD_CaptchaConfig->ImageHeight = 50;
In the code above, we have overridden the default settings of library for the reset password page. You can find a full list of available Captcha configuration options and related instructions at the Captcha configuration options page.
Please Note
The information on this page is out of date and applies to a deprecated version of BotDetect™ CAPTCHA (v3.0).
An up-to-date equivalent page for the latest BotDetect Captcha release (v4) is BotDetect v4 Captcha documentation index.
General information about the major improvements in the current BotDetect release can be found at the What's New in BotDetect v4.0 page.
Current BotDetect Versions
-
BotDetect ASP.NET CAPTCHA
2019-07-22v4.4.2 -
BotDetect Java CAPTCHA
2019-07-22v4.0.Beta3.7 -
BotDetect PHP CAPTCHA
2019-07-22v4.2.5